โ ๊ฐ ๊ฐ์ด ์ฝ๋ฉํด์ ์ ์น์ฒ๋ผ ์ฌํ์ด๋ค. โ
- ๊ฐ๋ฐ์ ์๋ด(Programmerโs Proverbs)
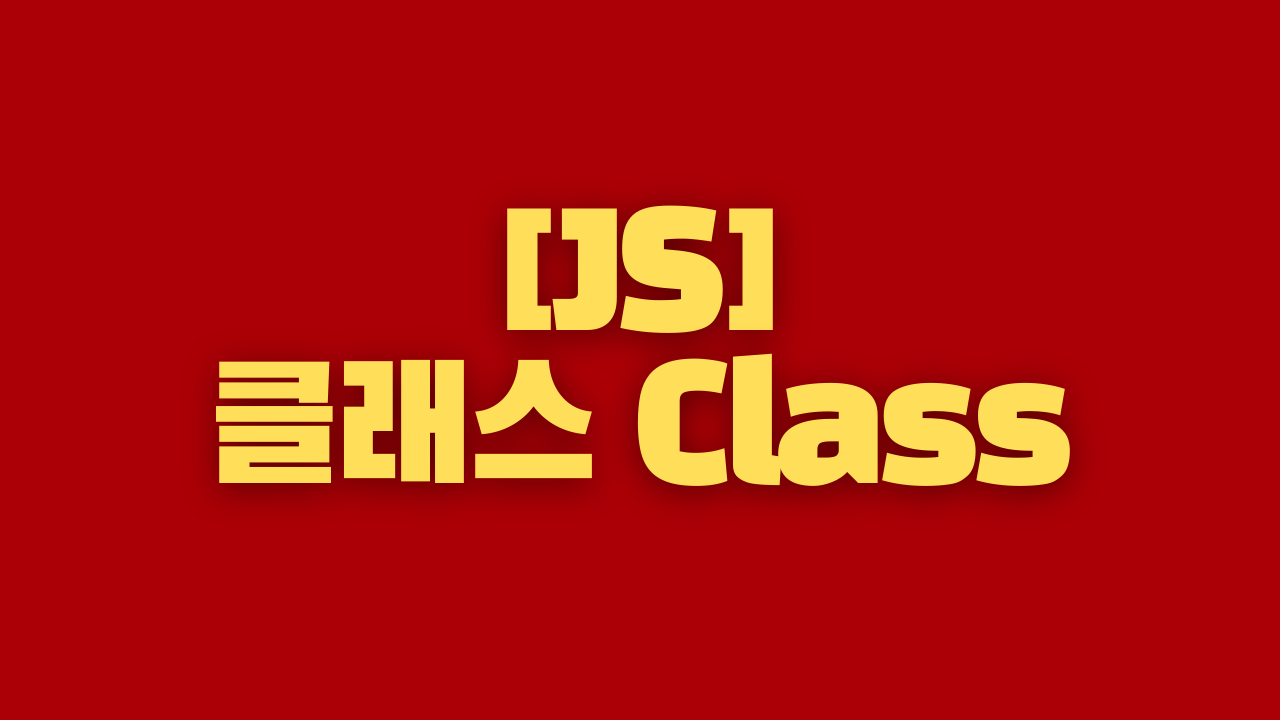
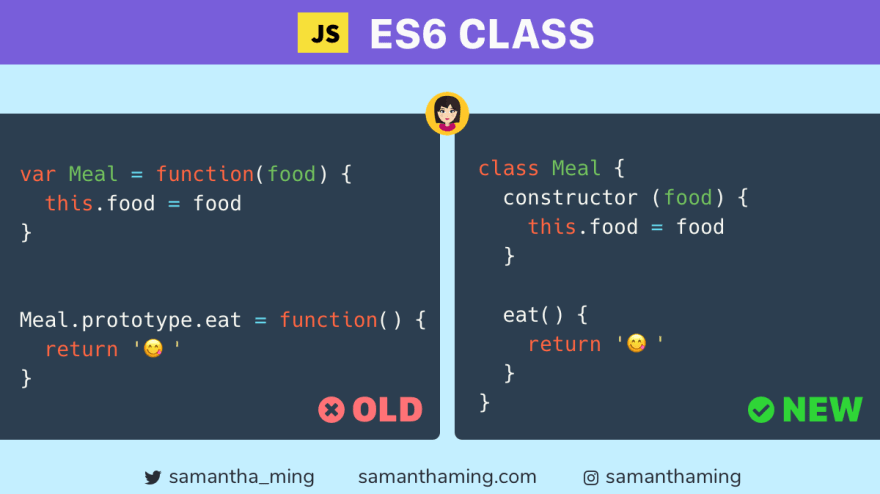
ES6 ํด๋์ค ๋ฌธ๋ฒ์ ์ข๋ JAVA์ค๋ฝ๊ฒ ๊ฐ์ฒด ์งํฅ์ ์ผ๋ก ํํํ๊ธฐ ์ํด ์ถ๊ฐ๋ ์๋ก์ด ๋ฌธ๋ฒ์ด๋ค.
ES5๊น์ง ์๋ฐ์คํฌ๋ฆฝํธ์๋ ํด๋์ค๊ฐ ์์๋ค. ๊ทธ๋์ ํ๋กํ ํ์ ์ฒด์ด๋์ ํตํด ํด๋์ค ๋น์ค๋ฌด๋ฆฌํ๊ฒ ๊ตฌํํด์์๋๋ฐ ES6 ๋ฒ์ ผ์ ๋ค์ด์๋ฉด์ ํด๋์ค์ ๋น์ทํ ๊ตฌ์กฐ ๋ฌธ๋ฒ์ ์ถ๊ฐํ์๋ค.
๋ค๋ง ์๊น์๋ง ํด๋์ค ๊ตฌ์กฐ์ด์ง, ์์ง ๋ด๋ถ์ ์ผ๋ก๋ ํ๋กํ ํ์ ๋ฐฉ์์ผ๋ก ์๋๋๋ค.
๋ค์์ ํ๋กํ ํ์ ๋ฌธ๋ฒ๊ณผ ํด๋์ค ๋ฌธ๋ฒ์ ๊ฐ๋จํ ์ฐจ์ด์ด๋ค.
์ด ๋์ ๊ฐ์ ๊ฒฐ๊ณผ๋ฅผ ์ถ๋ ฅํ์ง๋ง, ๋ฌธ๋ฒ ์๊น์๋ง ๋ค๋ฅด๊ณ ๋ด๋ถ ๋ก์ง์ ์์ ํ ๊ฐ์ ๊ตฌ์กฐ๋ผ๋ ์ ๋ง ๊ธฐ์ตํ๋ฉด ๋๋ค.
ES5 ํ๋กํ ํ์ ๋ฌธ๋ฒ
// ์์ฑ์
function Person({name, age}) {
this.name = name;
this.age = age;
}
Person.prototype.introduce = function() {
return `์๋
ํ์ธ์, ์ ์ด๋ฆ์ ${this.name}์
๋๋ค.`;
};
const person = new Person({name: '์ค์์ค', age: 19});
console.log(person.introduce()); // ์๋
ํ์ธ์, ์ ์ด๋ฆ์ ์ค์์ค์
๋๋ค.
โ
ES6 ํด๋์ค ๋ฌธ๋ฒ
// ํด๋์ค
class Person {
// ์ด์ ์์ ์ฌ์ฉํ๋ ์์ฑ์ ํจ์๋ ํด๋์ค ์์ `constructor`๋ผ๋ ์ด๋ฆ์ผ๋ก ์ ์ํฉ๋๋ค.
constructor({name, age}) { //์์ฑ์
this.name = name;
this.age = age;
}
// ๊ฐ์ฒด์์ ๋ฉ์๋๋ฅผ ์ ์ํ ๋ ์ฌ์ฉํ๋ ๋ฌธ๋ฒ์ ๊ทธ๋๋ก ์ฌ์ฉํ๋ฉด, ๋ฉ์๋๊ฐ ์๋์ผ๋ก `Person.prototype`์ ์ ์ฅ๋ฉ๋๋ค.
introduce() {
return `์๋
ํ์ธ์, ์ ์ด๋ฆ์ ${this.name}์
๋๋ค.`;
}
}
const person = new Person({name: '์ค์์ค', age: 19});
console.log(person.introduce()); // ์๋
ํ์ธ์, ์ ์ด๋ฆ์ ์ค์์ค์
๋๋ค.
์๋ฐ์คํฌ๋ฆฝํธ ํด๋์ค ๋ฌธ๋ฒ
Class ์ ์ธ
constructor๋ ์ธ์คํด์ค๋ฅผ ์์ฑํ๊ณ ํด๋์ค ํ๋๋ฅผ ์ด๊ธฐํํ๊ธฐ ์ํ ํน์ํ ๋ฉ์๋์ด๋ค.
constructor๋ ํด๋์ค ์์ ํ ๊ฐ๋ง ์กด์ฌํ ์ ์๋ค. 2๊ฐ ์ด์ ์์ ๊ฒฝ์ฐ Syntax Error๊ฐ ๋ฐ์ํ๋๊น ์ฃผ์ํ์.
class Person {
height = 180; // ์ธ์คํด์ค ๋ณ์
// constructor๋ ์ด๋ฆ์ ๋ฐ๊ฟ ์ ์๋ค.
constructor(name, age) {
// this๋ ํด๋์ค๊ฐ ์์ฑํ ์ธ์คํด์ค๋ฅผ ๊ฐ๋ฆฌํจ๋ค.
this.name = name;
this.age = age;
}
}
let person1 = new Person('john', 23);
console.log(person1.name); // john
console.log(person1.age); // 23
console.log(person1.height); // 180
ํด๋์ค ํ๋์ ์ ์ธ๊ณผ ์ด๊ธฐํ๋ ๋ฐ๋์ constructor ๋ด๋ถ์์ ์ค์ํ๋ค.
constructor ๋ด๋ถ์ ์ ์ธํ ํด๋์ค ํ๋๋ ํด๋์ค๊ฐ ์์ฑํ ์ธ์คํด์ค์ ๋ฐ์ธ๋ฉ ๋๋ค.
ํด๋์ค ํ๋๋ ๊ทธ ์ธ์คํด์ค์ ํ๋กํผํฐ๊ฐ ๋๋ฉฐ, ์ธ์คํด์ค๋ฅผ ํตํด ํด๋์ค ์ธ๋ถ์์ ์ธ์ ๋ ์ฐธ์กฐํ ์ ์๋ค. (public)
JAVA๋ Python์ ํด๋์ค๋ฌธ๋ฒ๊ณผ์ ์ฐจ์ด์ ์, ์๋ฐ์คํฌ๋ฆฝํธ์ ํด๋์ค ๋ฌธ๋ฒ์์ ์ธ์คํด์ค ๋ณ์๋ฅผ ๋ฐ๋์ ์ง์ ํ์ง ์๊ณ ์์ฑ์(constructor)์ ํตํด this.๋ณ์ ๋ฌธ๋ฒ์ผ๋ก ์๋ ์์ฑ๋ ์ ์๋ค๋ ์ ์ด๋ค.
ํด๋์ค์ ๋ณธ๋ฌธ(body)์ strict mode์์ ์คํ๋๋ค. ์ฑ๋ฅ ํฅ์์ ์ํด ๋ ์๊ฒฉํ ๋ฌธ๋ฒ์ด ์ ์ฉ๋๋ค.
Class ๋ฉ์๋ ์ ์
โํด๋์ค์ ๋ฉ์๋๋ฅผ ์ ์ํ ๋๋ ๊ฐ์ฒด ๋ฆฌํฐ๋ด์์ ์ฌ์ฉํ๋ ๋ฌธ๋ฒ๊ณผ ์ ์ฌํ ๋ฌธ๋ฒ์ ์ฌ์ฉํ๋ค.
class Calculator {
add(x, y) {
return x + y;
}
subtract(x, y) {
return x - y;
}
}
let calc = new Calculator();
calc.add(1,10); // 11
โ
๊ฐ์ฒด ๋ฆฌํฐ๋ด์ ๋ฌธ๋ฒ๊ณผ ๋ง์ฐฌ๊ฐ์ง๋ก, ์์์ ํํ์์ ๋๊ดํธ๋ก ๋๋ฌ์ธ์ ๋ฉ์๋์ ์ด๋ฆ์ผ๋ก ์ฌ์ฉํ ์๋ ์๋ค.
const methodName = 'introduce'; // ํด๋์ค ๋ฉ์๋ ์ด๋ฆ
class Person {
constructor({name, age}) {
this.name = name;
this.age = age;
}
// ์๋ ๋ฉ์๋์ ์ด๋ฆ์ `introduce`๊ฐ ๋ฉ๋๋ค.
[methodName]() {
return `์๋
ํ์ธ์, ์ ์ด๋ฆ์ ${this.name}์
๋๋ค.`;
}
}
console.log(new Person({name: '์ค์์ค', age: 19}).introduce()); // ์๋
ํ์ธ์, ์ ์ด๋ฆ์ ์ค์์ค์
๋๋ค.
โ
Getter / Setter
ํด๋์ค ๋ด์์ Getter ํน์ setter๋ฅผ ์ ์ํ๊ณ ์ถ์ ๋๋ ๋ฉ์๋ ์ด๋ฆ ์์ get ๋๋ set์ ๋ถ์ฌ์ฃผ๋ฉด ๋๋ค.
[JS] ๐ ์๋ฐ์คํฌ๋ฆฝํธ Getter & Setter ๊ฐ๋ ์ ๋ฆฌ
ํ๋กํผํฐ getter์ setter ๊ฐ์ฒด์ ํ๋กํผํฐ๋ ๋ ์ข ๋ฅ๋ก ๋๋ฉ๋๋ค. ์ฒซ ๋ฒ์งธ ์ข ๋ฅ๋ ๋ฐ์ดํฐ ํ๋กํผํฐ(data property) ์ ๋๋ค. ์ง๊ธ๊น์ง ์ฌ์ฉํ ๋ชจ๋ ํ๋กํผํฐ๋ ๋ฐ์ดํฐ ํ๋กํผํฐ์ ๋๋ค. ๋ ๋ฒ์งธ๋ ์ ๊ทผ
inpa.tistory.com
class Account {
constructor() {
this._balance = 0;
}
get balance() {
return this._balance;
}
set balance(newBalance) {
this._balance = newBalance;
}
}
const account = new Account();
account.balance = 10000;
account.balance; // 10000
โ
์ ์ ๋ฉ์๋ (static)
์ ์ ๋ฉ์๋๋ ํด๋์ค์ ์ธ์คํด์ค๊ฐ ์๋ ํด๋์ค ์ด๋ฆ์ผ๋ก ๊ณง๋ฐ๋ก ํธ์ถ๋๋ ๋ฉ์๋์ด๋ค.
static ํค์๋๋ฅผ ๋ฉ์๋ ์ด๋ฆ ์์ ๋ถ์ฌ์ฃผ๋ฉด ํด๋น ๋ฉ์๋๋ ์ ์ ๋ฉ์๋๊ฐ ๋๋ค.
์ฐ๋ฆฌ๊ฐ ๋๋ค๊ฐ์ ์ป๊ธฐ ์ํด Math.random() ๊ฐ์ ๋ฉ์๋๋ฅผ ์ฐ๋ฏ์ด, ๋ฐ๋ก new Math() ์์ด ๊ณง๋ฐ๋ก ํด๋์ค๋ช
.๋ฉ์๋๋ช
์ผ๋ก ํจ์๋ฅผ ํธ์ถํด์ ์ฌ์ฉํ๋ ๊ฒ์ด ๋ฐ๋ก radom ๋ฉ์๋๊ฐ static์ผ๋ก ์ค์ ๋์ด ์๊ธฐ ๋๋ฌธ์ด๋ค.
class Person {
constructor({ name, age }) { // ์์ฑ์ ์ธ์คํด์ค
this.name = name;
this.age = age;
}
static static_name = 'STATIC'; // ์ ์ ์ธ์คํด์ค
getName() { // ์ธ์คํด์ค(ํ๋กํ ํ์
) ๋ฉ์๋
return this.name;
}
static static_getName() { // ์ ์ ๋ฉ์๋
return this.static_name;
}
}
const person = new Person({ name: 'jeff', age: 20 });
person.getName(); // jeff
Person.static_getName(); // STATIC
class Person {
constructor({ name, age }) {
this.name = name;
this.age = age;
}
// ์ด ๋ฉ์๋๋ ์ ์ ๋ฉ์๋
static static_sumAge(...people) {
/*
ํจ์ ํ๋ผ๋ฏธํฐ people๋ฅผ ์ ๊ฐ์ฐ์ฐ์ ...people๋ฅผ ํตํด ๋ฐฐ์ด๋ก ๋ง๋ฌ
[ {"name": "์ค์์ค", age": 19}, { "name": "์ ํ๊ฒฝ","age": 20 }]
*/
// ๊ทธ๋ฆฌ๊ณ ๊ฐ ๊ฐ์ฒด์ age๊ฐ์ ์ป์ด์ ํฉ์นจ
return people.reduce((acc, person) => acc + person.age, 0);
}
}
const person1 = new Person({ name: '์ค์์ค', age: 19 });
const person2 = new Person({ name: '์ ํ๊ฒฝ', age: 20 });
Person.static_sumAge(person1, person2); // 39
โ
์ ๋๋ ์ดํฐ
Generator ๋ฉ์๋๋ฅผ ์ ์ํ๋ ค๋ฉด, ๋ฉ์๋ ์ด๋ฆ ์์ * ๊ธฐํธ๋ฅผ ๋ถ์ฌ์ฃผ๋ฉด ๋๋ค.
[JS] ๐ ์ ๋๋ ์ดํฐ - ์ดํฐ๋ ์ดํฐ ๊ฐํํ
์ ๋ค๋ ์ดํฐ๋? ์ดํฐ๋ฌ๋ธ์ด๋ฉฐ ๋์์ ์ดํฐ๋ ์ดํฐ = ์ดํฐ๋ ์ดํฐ๋ฅผ ๋ฆฌํดํ๋ ํจ์ (async๊ฐ Promise๋ฅผ ๋ฆฌํดํ๋ ํจ์๋ฏ์ด, ์ ๋๋ ์ดํฐ๋ ์ดํฐ๋ ์ดํฐ๋ฅผ ๋ฆฌํดํ๋ ํจ์๋ค.) โ ์ ๋๋ ์ดํฐ ํจ์๋ฅผ ์ฌ์ฉํ
inpa.tistory.com
class Gen {
*[Symbol.iterator]() {
yield 1;
yield 2;
yield 3;
}
}
// 1, 2, 3์ด ์ฐจ๋ก๋๋ก ์ถ๋ ฅ๋ฉ๋๋ค.
for (let n of new Gen()) {
console.log(n);
}
ํด๋์ค ์์ (Class Inheritance)
โํด๋์ค ์์(class inheritance, subclassing) ๊ธฐ๋ฅ์ ํตํด ํ ํด๋์ค์ ๊ธฐ๋ฅ์ ๋ค๋ฅธ ํด๋์ค์์ ์ฌ์ฌ์ฉํ ์ ์๋ค.
extends ํค์๋
extends ํค์๋๋ ํด๋์ค๋ฅผ ๋ค๋ฅธ ํด๋์ค์ ํ์ ํด๋์ค๋ก ๋ง๋ค๊ธฐ ์ํด ์ฌ์ฉ๋๋ค.
class Parent {
// ...
}
class Child extends Parent {
// ...
}
์ ์ฝ๋์์, extends ํค์๋๋ฅผ ํตํด Child ํด๋์ค๊ฐ Parent ํด๋์ค๋ฅผ ์์ํ๋ค.
์ด ๊ด๊ณ๋ฅผ ๋ณด๊ณ '๋ถ๋ชจ ํด๋์ค-์์ ํด๋์ค ๊ด๊ณ' ํน์ '์ํผ ํด๋์ค(superclass)-์๋ธ ํด๋์ค(subclass) ๊ด๊ณ'๋ผ๊ณ ๋งํ๊ธฐ๋ ํ๋ค.
โ๋ฐ๋ผ์ ์ด๋ค ํด๋์ค A๊ฐ ๋ค๋ฅธ ํด๋์ค B๋ฅผ ์์๋ฐ์ผ๋ฉด, ๋ค์๊ณผ ๊ฐ์ ์ผ๋ค์ด ๊ฐ๋ฅํด์ง๋ค.
- ์์ ํด๋์ค A๋ฅผ ํตํด ๋ถ๋ชจ ํด๋์ค B์ ์ ์ ๋ฉ์๋์ ์ ์ ์์ฑ์ ์ฌ์ฉํ ์ ์๋ค.
- ๋ถ๋ชจ ํด๋์ค B์ ์ธ์คํด์ค ๋ฉ์๋์ ์ธ์คํด์ค ์์ฑ์ ์์ ํด๋์ค A์ ์ธ์คํด์ค์์ ์ฌ์ฉํ ์ ์๋ค.
class Parent {
static staticProp = 'staticProp';
static staticMethod() {
return 'I\'m a static method.';
}
instanceProp = 'instanceProp';
instanceMethod() {
return 'I\'m a instance method.';
}
}
class Child extends Parent {}
// ์์ํ๋ฉด ๋ถ๋ชจ์ static์์๋ค์ ์ฌ์ฉ ๊ฐ๋ฅ
console.log(Child.staticProp); // staticProp
console.log(Child.staticMethod()); // I'm a static method.
// ์์ํ๋ฉด ๋ถ๋ชจ์ ์ธ์คํด์ค๋ฅผ ์ฌ์ฉ ๊ฐ๋ฅ
const c = new Child();
console.log(c.instanceProp); // instanceProp
console.log(c.instanceMethod()); // I'm a instance method.
super ํค์๋
โsuper ํค์๋์ ๋์ ๋ฐฉ์์ ๋ค์๊ณผ ๊ฐ๋ค.โ
- ์์ฑ์ ๋ด๋ถ์์ super๋ฅผ ํจ์์ฒ๋ผ ํธ์ถํ๋ฉด, ๋ถ๋ชจ ํด๋์ค์ ์์ฑ์๊ฐ ํธ์ถ
- ์ ์ ๋ฉ์๋ ๋ด๋ถ์์๋ super.prop๊ณผ ๊ฐ์ด ์จ์ ๋ถ๋ชจ ํด๋์ค์ prop ์ ์ ์์ฑ์ ์ ๊ทผํ ์ ์๋ค.
- ์ธ์คํด์ค ๋ฉ์๋ ๋ด๋ถ์์๋ super.prop๊ณผ ๊ฐ์ด ์จ์ ๋ถ๋ชจ ํด๋์ค์ prop ์ธ์คํด์ค ์์ฑ์ ์ ๊ทผํ ์ ์๋ค.
super(); // ๋ถ๋ชจ ์์ฑ์
super.๋ฉ์๋๋ช
// ์ ๊ทผ
class Person{
constructor(name, first, second){
this.name=name;
this.first=first;
this.second=second;
}
sum(){
return (this.first + this.second);
}
}
class Person2 extends Person{
// override Person
constructor(name, first, second, third){
super(name, first, second); //๋ถ๋ชจ ์์ฑ์๋ฅผ ๊ฐ์ ธ์์ ํํ๊ฒ ํ๋ค.
this.third = third;
}
sum(){
// ๋ถ๋ชจ ๋ฉ์๋๋ฅผ ๊ฐ์ ธ์์ ์ฌ์ฉ.
// ์ค๋ฒ๋ก๋ฉ ๋ฉ์๋์์ ์จ์ ํ ๋ถ๋ชจ ๋ฉ์๋๋ฅผ ์ฌ์ฉํ๊ณ ์ถ์๋
return super.sum() + this.third;
}
}
var kim = new Person2('kim', 10, 20, 30);
document.write(kim.sum()); // 60
Private ํด๋์ค ๋ณ์
์ด์ ๊น์ง ์๋ฐ์คํฌ๋ฆฝํธ ํด๋์ค์ ๋ชจ๋ ๋ฉ์๋๋ ํผ๋ธ๋ฆญ์ผ๋ก ์ง์ ๋์๋ค.
๊ทธ๋์ ์ ๋ช ๋ฌด์คํ ๋ฐ์ชฝ์ง๋ฆฌ ํด๋์ค ๊ตฌํ์ฒด๋ก ๋น๋์ ๋ฐ์์์ง๋ง, ES2021๋ถํฐ๋ ๋ฉ์๋์ ํ๋๋ช ์์ "#"์ ๋ถ์ฌ์ ํ๋ผ์ด๋น ๋ฉ์๋์ ํ๋ ์ ์๊ฐ ๊ฐ๋ฅํด์ก๋ค. (๋ณด๋ค JAVA ์ค๋ฌ์์ก๋ค)
# ๊ธฐํธ๋ฅผ ์ ๋์ฌ๋ก ์ฌ์ฉํ์ฌ ๋ฉ์๋์ ์ ๊ทผ์๋ฅผ ๋น๊ณต๊ฐ๋ก ์ค์ ํ ์ ์์ผ๋ฉฐ ๋์์ getter ๋ฐ setter ๋ฉ์๋๋ฅผ ์ฌ์ฉํ ์๋ ์๋ค.
class myClass {
// private ๋ณ์
#num = 100
// private ๋ฉ์๋
#privMethod(){
console.log(this.#num); // ํ๋ผ์ด๋น ๋ณ์ ํธ์ถ
}
publicMethod() {
this.#privMethod(); // ํ๋ผ์ด๋น ๋ฉ์๋ ํธ์ถ
}
}
let newClass = new myClass();
newClass.publicMethod() // 100
Private Fields ์ฒดํฌํ๊ธฐ
private ๋ณ์๋ฅผ ํด๋์ค์ ์ถ๊ฐํ๋ ๊ฒ ๊น์ง๋ ์ข์์ง๋ง, ํด๋์ค๋ฅผ ์ด์ฉํ ๋, ์ด ํด๋์ค ์ธ์คํด์ค๊ฐ private์ธ์ง public์ธ์ง ํ์ธ์ด ์ด๋ ค์ด ๊ฒฝ์ฐ๊ฐ ์์๋ค.
์๋ํ๋ฉด public ํ๋์ ๋ํด ํด๋์ค์ ์กด์ฌํ์ง ์๋ ํ๋์ ์ ๊ทผ์ ์๋ํ๋ฉด undefined๊ฐ ๋ฐํ๋๋ ๋ฐ๋ฉด์, private ํ๋๋ undefined๋์ ์์ธ๋ฅผ ๋ฐ์์ํค๊ฒ ๋๊ธฐ ๋๋ฌธ์ด๋ค. ๊ทธ๋์ ํน์ ๊ฐ์ฒด์ ์ด๋ค private ํ๋กํผํฐ๊ฐ ์๋์ง ํ์ธํ๊ธฐ ์ด๋ ค์ ๋ค.
๋ฐ๋ผ์ in ํค์๋๋ฅผ ์ฌ์ฉํด ์ด ๊ฐ์ฒด์์ private ์์ฑ/๋ฉ์๋ ๊ฐ ์๋์ง๋ฅผ ์ฒดํฌํ ์ ์๋ค.
class Foo {
#brand = 100;
static isFoo(obj) {
return #brand in obj;
}
}
const foo = new Foo();
const foo2 = { brand: 100 };
console.log('foo : ', Foo.isFoo(foo)); // true
console.log('foo2 : ', Foo.isFoo(foo2)); // false
ํด๋์ค ์์ & ํ๋กํ ํ์ ์์ ๋ฌธ๋ฒ ๋น๊ต
โํด๋์ค ์์์ ๋ด๋ถ์ ์ผ๋ก ํ๋กํ ํ์ ์์ ๊ธฐ๋ฅ์ ํ์ฉํ๊ณ ์๋ค.
์๋ ์ฝ๋์ ํด๋์ค ์์์ ๋ํ ํ๋กํ ํ์ ์ฒด์ธ์ ๊ทธ๋ฆผ์ผ๋ก ๋ํ๋ด๋ณด๋ฉด ๋ค์๊ณผ ๊ฐ์ด ๋๋ค.
class Person {}
class Student extends Person {}
const student = new Student();
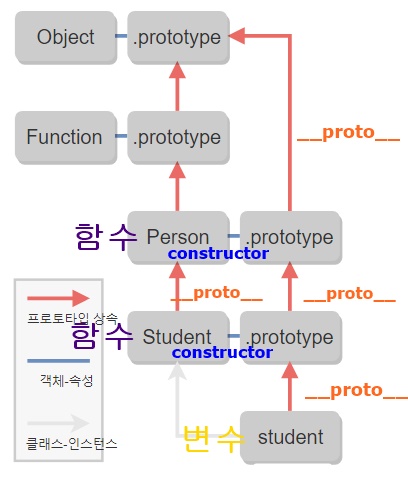
ํ๋กํ ํ์ ์์ ๋ฐฉ์
//ํ๋กํ ํ์
๋ฐฉ์
function Person(name, first, second){
this.name=name;
this.first=first;
this.second=second;
}
Person.prototype.sum = function() {
return (this.first + this.second);
}
function Student(name, first, second, third){
Person.call(this, name, first, second);
//Person์ ์์ฑ์ ํจ์์ด๋ค. ๊ทธ๋ฅ ์ฐ๋ฉด this๋ ๋ญ๋ฐ? ๊ทธ๋์ ์ด๋ call,bindํจ์๋ฅผ ์จ์ค๋ค.
//ํด๋์ค์ super() ์ ๊ฐ์ ์ญํ ์ ํ๋ค๊ณ ๋ณด๋ฉด ๋๋ค. ํ์ง๋ง ์์ง ์์์ด ๋๊ฑด ์๋๋ค.
this.third = third;
}
//๋ฐฉ๋ฒ 1 ๋นํ์ค :
//Student ํ๋กํ ํ์
์ค๋ธ์ ํธ ๋งํฌ๋ฅผ Person ํ๋กํ ํ์
์ค๋ธ์ ํธ๋ก ์ฐ๊ฒฐ์์ผ sum์ ์ฌ์ฉํ ์์๊ฒ ์ฐพ์๊ฐ๋๋ก ์ค์
Student.prototype.__proto__ = Person.prototype;
//๋ฐฉ๋ฒ 2 ํ์ค :
//Personํ๋กํ ํ์
์ค๋ธ์ ํธ๋ฅผ ์๋ก ๋ง๋ค์ด์ ๋์
Student.prototype = Object.create(Person.prototype);
Student.prototype.constructor = Student; //์์ฑ์ ์ฐ๊ฒฐ
Student.prototype.avg = function(){
return (this.first+this.second+this.third)/3;
}
var kim = new Student('kim', 10, 20, 30);
ํด๋์ค ์์ ๋ฐฉ์
//ํด๋์ค ๋ฐฉ์
class Person{
constructor(name, first, second){
this.name=name;
this.first=first;
this.second=second;
}
sum(){
return (this.first + this.second);
}
}
class Student extends Person{
constructor(name, first, second, third){
super(name, first, second);
this.third = third;
}
sum(){
return super.sum() + this.third;
}
avg(){
return (this.first+this.second+this.third)/3;
}
}
var kim = new Student('kim', 10, 20, 30);
โ
์ด ๊ธ์ด ์ข์ผ์ จ๋ค๋ฉด ๊ตฌ๋ & ์ข์์
์ฌ๋ฌ๋ถ์ ๊ตฌ๋
๊ณผ ์ข์์๋
์ ์์๊ฒ ํฐ ํ์ด ๋ฉ๋๋ค.